Introduction
Your program is to use the brute-force approach in order to find the Answer to Life, the Universe, and Everything. More precisely... rewrite small numbers from input to output. Stop processing input after reading in the number 42. All numbers at input are integers of one or two digits.
Input:
1
2
88
42
99
Output:
1
2
88
Approach
The approach could be to have a loop that breaks when the input number is 42.
//Rextester.Program.Main is the entry point for your code. Don't change it.
//Microsoft (R) Visual C# Compiler version 2.9.0.63208 (958f2354)
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text.RegularExpressions;
namespace Rextester
{
public class Program
{
public static void Main(string[] args)
{
const int number = 42;
var input = 0;
while (input != number) {
var inputStr = Console.ReadLine();
if (int.TryParse(inputStr, out input) && input != number) {
Console.WriteLine(input);
}
}
}
}
}
Reference
SPOJ.com - Problem TEST
...
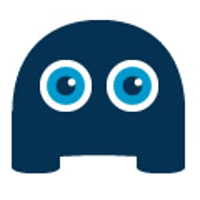
TEST - Life, the Universe, and Everything, C# - rextester